1. cookie의 개념
# cookie
: 서버가 사용자의 웹 브라우저에 전송하는 작은 데이터 조각
- 브라우저는 그 데이터 조각들을 저장해 놓았다가, 동일한 서버에 재 요청 시 저장된 데이터를 함께 전송
- 쿠키는 두 요청이 동일한 브라우저에서 들어왔는지 아닌지를 판단할 때 주로 사용
- 사용자의 로그인 상태를 유지 가능
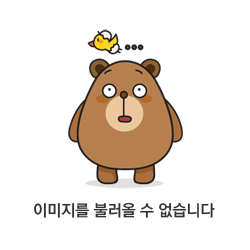
2. 접속할 때마다 count 증가하기
# cookie-parser
: 요청된 쿠키를 쉽게 추출할 수 있도록 도와주는 미들웨어
▼ 설치 및 사용방법
cookie-parser
Parse HTTP request cookies. Latest version: 1.4.6, last published: a year ago. Start using cookie-parser in your project by running `npm i cookie-parser`. There are 7875 other projects in the npm registry using cookie-parser.
www.npmjs.com
- app_cookie.js
<javascript />
const express = require('express');
const cookieParser = require('cookie-parser')
const app = express();
app.use(cookieParser());
app.get('/count', (req,res)=>{
if(req.cookies.count){
var count = parseInt(req.cookies.count);
}else{
var count = 0;
}
count = count + 1;
res.cookie('count',count);
res.send('count : '+ count);
});
app.listen(3003, ()=>{
console.log('Connected 3003 port!!');
});
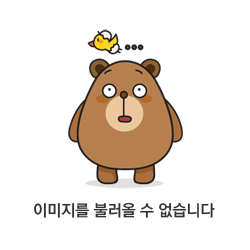
3. 간단한 쇼핑카트 프로그램
- /products 에 접속
- product 화면에서는 구매 상품 목록과 add 버튼 출력
- 각 상품의 add 선택시 카트에 추가되며 카트 화면으로 이동
- cart 화면에서는 구매한 상품의 이름과 수량 출력
- 서버에 재접속해도 카트 내용은 그대로 저장
- 카트에 대한 정보는 cookie로 저장하며 암호화할 것
![]() |
![]() |
<javascript />
const express = require('express');
const cookieParser = require('cookie-parser')
const app = express();
app.use(cookieParser('37832782@322!%^*'));
var products = {
1: { title: 'The history of web 1' },
2: { title: 'The next web' }
};
app.get('/products', (req, res) => {
var output = '';
for (var name in products) {
output += `
<li>
${products[name].title}<a href="/cart/${name}"> add</a>
</li>`;
}
res.send(`<h1>Products</h1><ul>${output}</ul><a href="/cart">Cart</a>`);
});
app.get('/cart/:id', (req, res) => {
var id = req.params.id;
if (req.signedCookies.cart) {
var cart = req.signedCookies.cart;
} else {
var cart = {};
}
if (!cart[id]) {
cart[id] = 0;
}
cart[id] = parseInt(cart[id]) + 1;
res.cookie('cart', cart,{signed:true});
res.redirect('/cart');
});
app.get('/cart', (req, res) => {
var cart = req.signedCookies.cart;
if (!cart) {
res.render('Empty!');
} else {
var output = '';
for (var id in cart) {
output += `<li>${products[id].title} (${cart[id]})</li>`;
}
}
res.send(`<h1>Cart</h1><ul>${output}</ul><a href = "/products">Products List</a>`);
});
app.listen(3003, () => {
console.log(`connected 3003 port!!`);
});
'BACKEND > Node.js' 카테고리의 다른 글
[ 생활코딩 - Node.js 활용하기] 03. Security Password (1) | 2022.12.01 |
---|---|
[ 생활코딩 - Node.js 활용하기] 2. Session (0) | 2022.11.28 |
[코드잇 - Node.js 기본기] 4. 서드파티 모듈과 npm 제대로 배우기 (1) | 2022.11.26 |
[코드잇 - Node.js 기본기] 3. 초간단 웹서버 만들기 (1) | 2022.11.26 |
[코드잇 - Node.js 기본기] 2. Node.js 기본 개념 - ② 비동기 프로그래밍 (0) | 2022.11.26 |